Regression and Gradient Descent
1 | import numpy as np |
1 | data = np.genfromtxt("gradient-descent-data.csv", delimiter=",") |
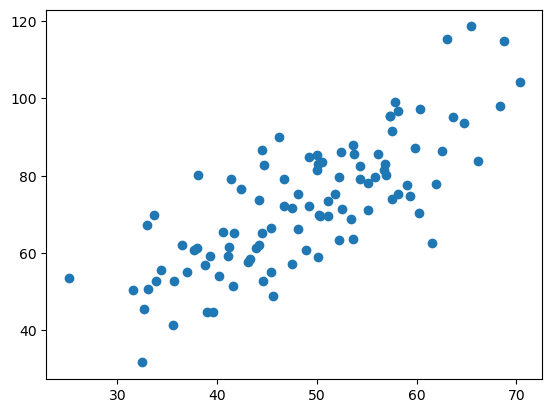
1 | # lost function |
1 | # lr learning rate |
The output result:
1 |
|
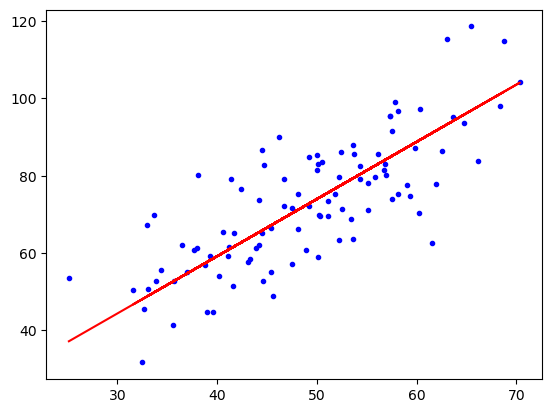
Sklearn
1 | from sklearn.linear_model import LinearRegression |
1 | data = np.genfromtxt("gradient-descent-data.csv", delimiter=",") |
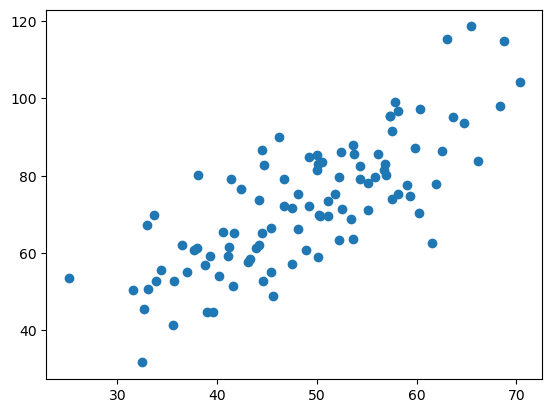
(100,)
1 | x_data = data[:, 0, np.newaxis] |
LinearRegression()In a Jupyter environment, please rerun this cell to show the HTML representation or trust the notebook.
On GitHub, the HTML representation is unable to render, please try loading this page with nbviewer.org.
LinearRegression()
1 | plt.plot(x_data,y_data,'b.') |
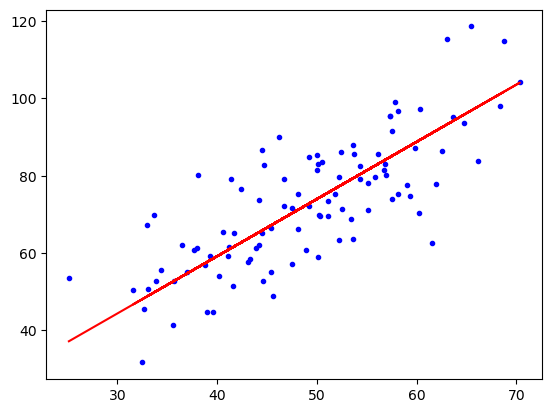